From time to time I am asked to advise a good administration and development tool for MySQL.
Of course, there is an official MySQL GUI Tools Bundle for 5.0. But is it the best option to manage MySQL database? Unfortunately, I doubt it.
For instance, I have found three much more interesting tools: SQLyog, EMS MySQL Manager and Toad for MySQL.
SQLyog and EMS MySQL Manager are good tools unless you want to save your budget for something else. It shouldn’t be a big deal for business but for an independent developer could be quite important.
So the best option in my opinion is Toad for MySQL. Granted it has everything required for database development (basically as any other mentioned above tool) but it also has perfect user interface and ‘look and feel’. You will understand that especially if you had to switch to MySQL development after experience with either MS Query Analyzer or MS SQL Server Management Studio.
There is also EMS MySQL Manager Lite which is freeware too. But it has limits on database size as well as all concept of EMS user interface ‘logic’ is completely different which can be uncomfortable for many developers. But also keep in mind that EMS releases comprehensive MySQL products family.
For the moment I cannot advise any tool for Mac or Linux but phpMyAdmin will work for 100% 😉 .
Below is Toad for MySQL screenshot.
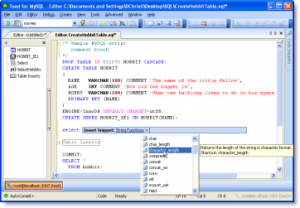
P.S.: I am still looking for anything worthy for MySQL routines development and version control. I.e. something similar or better than MS Visual Studio Database Projects. There was no sense of such tools before since MySQL did not support stored procedures, functions and views. But now it would be good to have tools for database code management.